关键词 >
MPSC/Mathematics 451 Assignment Four–Part One
发布时间:2020-10-13
Fall 2017
You are to write a MATLAB function to find the cube root of a complex number.
In general, a complex number z is represented as

where z1 and z2 are real numbers and i is the imaginary unit. In MATLAB (or Octave), you can compos z out of x and y from the statement
z = complex(z1, z2)

where z is described in (1). You are to use this function to compute the cube root of a using Newton’s method for two variables.
To do that, we represent the complex numbers z and a as vectors with two real components. That is the vectors
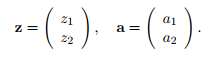
represent z in (1) and a = a1 + ia2.
Then we write f(z) as a function of the two real numbers z1 and z2 parameterized by the two real numbers a1 and a2. Equation (2) my be written
f(z; a) = g1(z1, z2; a) + ig2(z1, z2; a) = 0.
where g1(·) and g2(·) are real valued functions. For f(z) to be zero, both g1(z1, z2) and g2(z1, z2) must be zero since the real and imaginary parts separate. If we let

then
G(z; a) = 0
is equivalent to (2).
The first thing you need to do in this assignment is to identify g1(z1, z2; a) and g2(z1, z2; a) to create the function Gcubrt(z, a) and implement it in MATLAB. You then need to compute the Jacobian

and create the matrix valued function Jcubrt(z) in MATLAB.
Using these two functions, create a MATLAB function with the first
three lines are
function [z, niter] = ComCubrt(a)
avec=[real(a); imag(a)]; % Represent the complex number a as the vector avec
zvec=avec; % Just make a the initial guess for its cube root
Here a is a complex number and z is one of its cube roots. The parameter niter is the number of iterations required. ComCubrt(a) finds z from a using Newton’s method for two variables.
The last line of ComCubrt should be
z = complex(zvec(1), zvec(2));
so that you can return a complex number z as your answer.
Your iteration will produce a sequence of 2-vectors z(0) = a, z(1), . . . , which terminates when
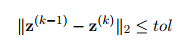
or
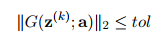
where

and ✏ is the value from the MATLAB eps command. For debugging reason, do no more than 25 iterations. Of course, do not keep all of the iterates z(k), just keep the two most recent ones so that you implement the termination criteria.
Use your routine to find the cube roots of a = 3 + 4i, 10 " 5i, "1 + 2i.
You are to write a MATLAB function to find the cube root of a complex number.
In general, a complex number z is represented as

where z1 and z2 are real numbers and i is the imaginary unit. In MATLAB (or Octave), you can compos z out of x and y from the statement
z = complex(z1, z2)
and recover z1 and z2 from z using
Given the complex number a = a1 + ia2, we are looking for a root of |

where z is described in (1). You are to use this function to compute the cube root of a using Newton’s method for two variables.
To do that, we represent the complex numbers z and a as vectors with two real components. That is the vectors
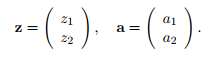
represent z in (1) and a = a1 + ia2.
Then we write f(z) as a function of the two real numbers z1 and z2 parameterized by the two real numbers a1 and a2. Equation (2) my be written
f(z; a) = g1(z1, z2; a) + ig2(z1, z2; a) = 0.
where g1(·) and g2(·) are real valued functions. For f(z) to be zero, both g1(z1, z2) and g2(z1, z2) must be zero since the real and imaginary parts separate. If we let

then
G(z; a) = 0
is equivalent to (2).
The first thing you need to do in this assignment is to identify g1(z1, z2; a) and g2(z1, z2; a) to create the function Gcubrt(z, a) and implement it in MATLAB. You then need to compute the Jacobian

and create the matrix valued function Jcubrt(z) in MATLAB.
Using these two functions, create a MATLAB function with the first
three lines are
function [z, niter] = ComCubrt(a)
avec=[real(a); imag(a)]; % Represent the complex number a as the vector avec
zvec=avec; % Just make a the initial guess for its cube root
Here a is a complex number and z is one of its cube roots. The parameter niter is the number of iterations required. ComCubrt(a) finds z from a using Newton’s method for two variables.
The last line of ComCubrt should be
z = complex(zvec(1), zvec(2));
so that you can return a complex number z as your answer.
Your iteration will produce a sequence of 2-vectors z(0) = a, z(1), . . . , which terminates when
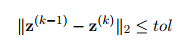
or
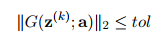
where

and ✏ is the value from the MATLAB eps command. For debugging reason, do no more than 25 iterations. Of course, do not keep all of the iterates z(k), just keep the two most recent ones so that you implement the termination criteria.
Use your routine to find the cube roots of a = 3 + 4i, 10 " 5i, "1 + 2i.