关键词 > CSSE7030
CSSE7030 Into The Breach (ish) Assignment 2 Semester 1, 2024
发布时间:2024-05-17
Hello, dear friend, you can consult us at any time if you have any questions, add WeChat: daixieit
Into The Breach (ish)
Assignment 2
Semester 1, 2024
CSSE7030
Due date: 24 May 2024, 16:00 GMT+10
1 Introduction
In this assignment, you will implement a (heavily) simplified version of the video game ”Into The Breach”. In this game players defend a set of civilian buildings from giant monsters. In order to achieve this goal, the player commands a set of equally giant mechanical heroes called ”Mechs” . There are a variety of enemy and mech types, which each behave slightly differently. Gameplay is described in section 3 of this document.
Unlike assignment 1, in this assignment you will be using object-oriented programming and following the Apply Model-View-Controller design pattern shown in lectures. In addition to creating code for modelling the game, you will be implementing a graphical user interface (GUI). An example of a final completed game is shown in Figure 1.
2 Getting Started
Download a2. zip from Blackboard — this archive contains the necessary files to start this assign- ment. Once extracted, the a2. zip archive will provide the following files:
a2. py This is the only file you will submit and is where you write your code. Do not make changes to any other files.
a2 support. py Do not modify or submit this file, it contains pre-defined classes, functions, and con- stants to assist you in some parts of your assignment. In addition to these, you are encouraged to create your own constants and helper functions in a2. py where possible.
levels/ This folder contains a small collection of files used to initialize games of Into The Breach. In addition to these, you are encouraged to create your own files to help test your implementation where possible.
3 Gameplay
This section describes an overview of gameplay for Assignment 2. Where interactions are not ex- plicitly mentioned in this section, please see Section 4.
3.1 Definitions
Gameplay takes place on a rectangular grid of tiles called a board, on which different types of entities can stand. There are three types of tile: Ground tiles, mountain tiles, and building tiles. Building
Figure 1: Example screenshot from a completed implementation. Note that your display may look slightly different depending on your operating system.
tiles each possess a given amount of health, which is the amount of damage they can suffer before they are destroyed. A building is destroyed if its health drops to 0. A tile may be blocking, in which case entities cannot stand on it. Tiles that are not blocking may have a maximum of one entity standing on them at any given time. Ground tiles are never blocking, mountain tiles are always blocking, and building tiles are blocking if and only if they are not destroyed.
Entities may either be Mechs, which are controlled by the player, or Enemies, which attack the player’s mechs and buildings. There are two types of mech; the Tank Mech and the Heal Mech. There are also two types of enemy; the Scorpion and the Firefly. Each entity possesses 4 characteristics:
1. position: the coordinate of the tile within the board on which the entity is currently standing.
2. health: the remaining amount of damage the entity can suffer before it is destroyed. An entity is destroyed the moment its health drops to 0, at which point it is immediately removed from the game.
3. speed: the number of tiles the entity can move during its movement phase (see below for details). Entities can only move horizontally and vertically; that is, moving one tile diagonally is considered two individual movements.
4. strength: how much damage the entity deals to buildings and other entities (i.e. the amount by which it reduces the health of attacked buildings or entities).
The game is turn based, with each turn consisting of a player movement phase, an attack phase, and an enemy movement phase. During the player movement phase, the player has the option to move each of the mechs under their control to a new tile on the grid. During the attacking phase, each mech and enemy perform an attack: an action that can damage mechs, enemies, or even buildings. Each enemy, mech, and building can only receive a certain amount of damage. If a mech or enemy is destroyed before they attack during a given attack phase, they do not attack during that attack phase. During the enemy movement phase, each enemy chooses a tile as their objective, and then moves to a new tile on the grid such that they are closer to their objective. The order in which mechs and enemies move and attack is determined by a fixed priority that will be displayed to the user at all times.
A valid path within the board is a sequence of movements into vertically or horizontally adjacent non-blocking tiles which do not contain an entity. The length of a valid path is the number of movements made within it. Note that each entity can only move through valid paths of length less than or equal to their maximum path length (speed).
A game of Into The Breach is over when either:
1. The player wins because at the end of an attack phase, all enemies are destroyed, at least one mech is not destroyed, and at least one building on the board is not destroyed.
2. The player loses because at the end of an attack phase, all buildings on the board are destroyed, or all mechs are destroyed.
3.2 Game phases
The game begins with a board of tiles, with entities occupying non-blocking tiles (at least one mech and at least one enemy). The exact set of tiles and entities is given by the level file used to initialise the game. Next to the board of tiles, a list is presented. Each element of the list displays an entity, alongside its position, current health, and current strength. The list is ordered by entity priority, with the highest priority entity appearing at the top (see Figure 1 for an example).
The following four phases repeat until the end of the game:
1. Player movement phase: This is the main phase of the game where all user interaction occurs. The user may click on any tile on the board. The action taken after a tile is clicked is sum- marized in Table 1. See Figure 2 for an example of the movement system. During the player movement phase, the user may also click one of the three buttons:
❼ If the user clicks the Save button, they should be prompted to enter a name for their save file via a filedialog. Upon entering a name and clicking to save the file, a new level file should be created based on the current game state. If a mech has been moved before the save button is clicked, the user is warned instead via an error message box.
❼ If the user clicks the Load button they should be prompted to select a saved file with a filedialog. When they select a file gameplay should restart as if the selected level file was the file used to initialise the game.
❼ If the user clicks the Undo button, the most recent move made by the user during the current player movement phase is reverted
❼ If the user clicks the End Turn button, the current player movement phase is ended, and the program moves onto the attack phase.
2. Attack phase: During the attack phase each entity, in descending order of priority, makes an attack. An attack affects a certain set of tiles depending on the entity making it. See Table 2 for the tiles affected by each entity. If a building tile is affected by an attack, then that building loses health equal to the strength of the attacking entity. If an entity is on a tile affected by an attack, then that entity is affected in a manner depending on what entity is performing the attack. See Table 2 for the effects of attacks for each entity. If an entity is destroyed during the attack phase by an entity with higher priority, it does not attack and is removed from the game. After each entity has performed an attack, the program immediately moves to the enemy movement phase.
3. Enemy movement phase: During the enemy movement phase, all enemies are assigned an objective. An objective is the position of a tile on the board and is assigned based on the type of entity as described in Table 3. Each enemy, in descending priority order, then moves to the tile that minimizes the length of the shortest path from itself to it’s objective. Note that the enemy can only move to tiles reachable via valid paths of length no greater than it’s speed. If there exists no valid path from an enemy to its objective, the enemy does not change position. After every enemy has moved, the display is updated and the program moves to termination checking.
4. Termination checking: If all enemies are destroyed, at least one mech is not destroyed, and at least one building on the board is not destroyed, the user has won and a victory message is displayed via an info messagebox. If all buildings on the board are destroyed or all mechs are destroyed, the user has lost and a defeat message is displayed via an info messagebox. Both victory and defeat messageboxes ask the user if they wish to play again. If the user does want to play again, then the game is reinitialised using the level file and gameplay starts again from the beginning. If the user does not want to play again the program closes the game window and exits gracefully. If no messageboxes were displayed then the program immediately returns to the player movement phase.
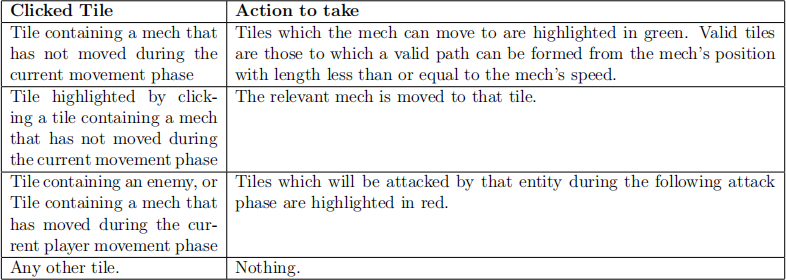
Table 1: Effect of clicking tiles during player movment phase. Every time the user clicks a tile, all previous highlighting is removed.
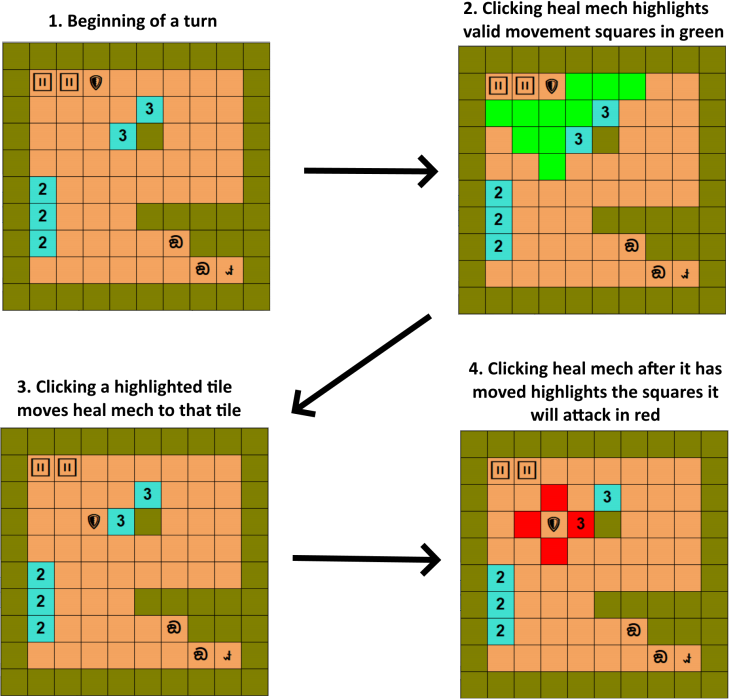
Figure 2: Movement of a mech during the player movement phase. The user clicks on the Heal Mech, and then clicks on one of the highlighted squares. Clicking the heal mech again highlights the squares it will attack.
Tiles Affected |
Attack Effect |
|
Tank Mech |
The two sets of five tiles extend- ing in a horizontal line from the tank mech: beginning from the tile directly left of the tank mech and extending left, and beginning from the tile directly right of the tank mechand extending right re- spectively. |
Receive damage equal to strength of tank mech. |
Heal Mech |
The four tiles directly adjacent to heal mech (not including diago- nals) |
If target isamech, recover health equal to strength of heal mech. Do nothing otherwise. |
Scorpion |
The four sets of two tiles ex- tending in horizontal and verti- cal lines from the scorpion: be- ginning from the tile directly left of the scorpion and extending left, beginning from the tile directly right of the scorpion and extend- ing right, beginning from the tile directly above of the scorpion and extending upward, and beginning from the tile directly below scor- pion and extending downwards respectively. |
Receive damage equal to strength of scorpion. |
Firefly |
The two sets of five tiles extend- ing in a vertical line from the fire- fly: beginning from the tile di- rectly above of the firefly and ex- tending upwards, and beginning from the tile directly below the firefly and extending downwards respectively. |
Receive damage equal to strength of firefly. |
Table 2: Entity attack behavior
Assigned Objective |
|
Scorpion |
Position of tile containing mech with the greatest health. If two mechs are tied for greatest health, choose position of tile containing the mech with the highest priority. |
Firefly |
Position of building tile with the least health amongst the buildings that are not destroyed. If two buildings are tied for the least health, choose the position of the building tile in the bottommost row. If there is still a tie for lowest health, choose the position of the building tile in the rightmost column. |
Table 3: Enemy objectives