关键词 > COMP3023
COMP 3023 – Design Patterns with C++ Assignment 2: Patient Vitals Management System.
发布时间:2024-05-15
Hello, dear friend, you can consult us at any time if you have any questions, add WeChat: daixieit
COMP 3023 – Design Patterns with C++
Assignment 2: Patient Vitals Management System.
Weighting: 20%,
Due Date: See course website
Problem statement
In this individual assignment, you have been tasked by your client to complete a patient management system. An increase in the occurrences of diseases once thought fictional (Amogus sus, E Rush, Nocap Syndrome, and Advanced Ticctocc Brain Damage) have made this project of utmost urgency. The patient management system should record patient vitals (heart rate, oxygen saturation, body temperature, and brain activity) and report alerts for concerning results dependent on the disease they have.
The system should load a list of patients. Currently this occurs from a database (mocked for this assignment), but you will change it to support loading from a file. Patients have vitals that are periodically measured. The system should raise an alert level on the patient when vital are recorded that exceed certain limits dependent on the disease they have. Use the following table to determine how to calculate the alert level:
Table 1: Alert level calculations
Disease |
Vitals |
Alert level |
Amogus sus |
Heart rate (HR) > 200 Heart rate (HR) > 210 Heart rate (HR) > 220 |
YELLOW ORANGE RED |
E Rush |
Body temperature (BT) > 38 and brain activity (BA) > 100 Body temperature (BT) > 38 and brain activity (BA) > 110 |
YELLOW RED |
Nocap Syndrome |
Oxygen saturation (SP02) < 94 Oxygen saturation (SP02) < 92 Oxygen saturation (SP02) < 90 |
YELLOW ORANGE RED |
Ticctocc Brain |
Age < 35 and brain activity (BA) < 10 |
RED |
Damage |
Age >= 35 and brain activity (BA) < 20 |
RED |
The alert levels are ranked ordered as Green, Yellow, Orange, Red, with Green being the lowest alert level. A patient defaults Green alert level unless their vitals raise their level.
Assignment overview
Part of the project has already been developed. You must adapt and buildupon the provided project. This requires not only understanding the existing codebase and writing new code, but also understanding the existing design and being able to explain your design changes. Read through this entire assignment specification before you begin, as you are required to explain many aspects of what you have done later in the assignment.
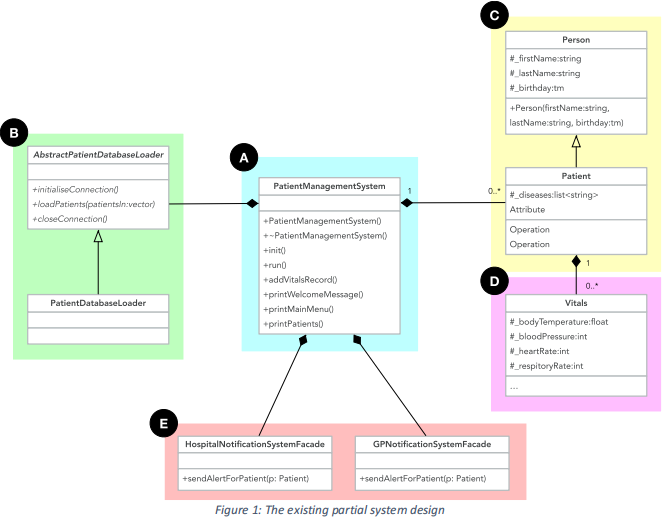
The existing system in presented in Figure 1. The main driver of the program is the Patient Management System class A. The system can currently connect to a patient database (mocked for this assignment) using B and load patients into the system. Patients are represented by the Patient class C. The system can currently record patient Vitals D through a command line interface. There is little error checking on this interface —we can presume the user will always enter the correct input. Lastly, Façade interfaces have been provided to notify Hospitals and GPs of any patient Alert Levels of concern E, though these still need to be integrated into the system process.
In this assignment, you will address functional requirements, non-functional requirements, and document your design and implementation. There are four major functional requirements you must address (FR1– FR4). These requirements will require you to write correct algorithms in C++ to identify patient alert levels when they record new Vitals measures (FR1), calculate and select the highest alert level for patients with multiple diseases (FR2), load patients from a file (FR3), and alert the hospitals and GPs when a patient has a Red alert level (FR4). Then you will present your design and implementation for each of these requirements in the form of a design document.
You must implement four design patterns throughout this assignment:
• Adapter pattern
• Composite pattern
• Observer pattern
• Strategy pattern
Each of these patterns will map to one of the major functional requirements of this assignment.
Each of the functional requirements arepresented in the followingAssignment
Requirements Taskssection, along with a description of the required documentation. The sectionGeneral requirementsthen describes the general requires that must be adhered to throughout the assignment.
Learning outcomes
After completing this assignment, you will have learnt to:
• Apply design patterns to solve design problem (CO2, CO2).
• Modify and expand a system in C++ using an object-oriented design (CO2, CO3).
• Write C++ code using modern C++ memory management (CO4).
• Write safe C++ code (CO4).
Assignment Requirements Tasks
This section presents the specific requirements you must address to complete the assignment.
Functional requirement FR1: Calculate the patient alert levels
The system should use the appropriate algorithm for calculating a patient’s Alert Level dependant on the patient’sprimary disease:
• Each patient will use an algorithm dependant on their primary disease. Reference Table 1 when writing your algorithms.
• The alert level must be calculated whenever new Vitals are recorded for a patient.
• The alert level must not be calculated for historical patient data loaded from a database or file.
Complete these steps:
1. Run the current system and record a Vitals for a patient. This will help you understand the current functionality.
2. Consider what design pattern can be used to address this problem.
3. Modify the design class diagram to factor in the pattern.
4. Implement the design changes to address the problem.
Functional requirement FR2: Calculate the alert level for all diseases a patient has
A patient may have more than one disease. The system should calculate a patient’s highest Alert Level dependent on all their diseases:
• Each patient will use all algorithms applicable to their diseases. Reference Table 1 when writing your algorithms.
• The final Alert Level must be the highest level of alert calculated for all their diseases.
• The alert level must be calculated whenever new Vitals are recorded for a patient.
• The alert level must not be calculated for historical patient data loaded from a database or file.
Complete these steps:
1. Consider what design pattern can be used to address this problem.
2. Modify the design class diagram to factor in the pattern.
3. Implement the design changes to address the problem.
Functional requirement FR3: Load patients from file
The system should load patient data from a file:
• You must use the PatientFileLoader class to load patient data from the file.
• The PatientFileLoader class is incomplete; you must complete it to correctly load from the file.
• Do not change the interface of the PatientFileLoader (i.e. the header file).
• Implementing this into the design must use the AbstractPatientDatabaseLoader interface.
• A file is provided (patients.txt) that contains the patients to load.
• The new loader can replace the existing loader specified in the
PatientManagementSystem, but it should be trivial to switchback to the original method (e.g. one line of code change).
• You will not need to use the PatientDatabaseLoader again in this assignment.
Complete these steps:
1. Consider what design pattern can be used to address this problem.
2. Modify the design class diagram to factor in the pattern.
3. Complete the PatientFileLoader so that it loads the patient data from file.
4. Implement the design changes to address the problem.
Parsing tips
• Re-watch week 6 lecture to make sure you master streams
• Testing for stream.eof() to check for the end of a stream is sometimes unreliable. Try using stream.peek(), which returns -1 when the end has been reached.
• You can read until a specified delimiter using std::getline bypassing the delimiter character as 3rd argument. For instance, std::getline(stream, destString,
'/') will read until the next slash character.
Functional requirement FR4: Alert the hospitals and GPs
The system should notify stakeholders when a patient’s alert level reaches Red:
• Façade interfaces (HospitalNotificationSystemFacade and
GPNotificationSystemFacade) are provided that can alert hospitals and GPs of concerning patients. You need to connect these facades to changes inpatient alert levels.
• This notification should happen immediately when a patient’s Alert Level changes.
Complete these steps:
1. Consider what design pattern can be used to address this problem.
2. Modify the design class diagram to factor in the pattern.
3. Implement the design changes to address the problem.
Non-functional requirement NFR1: Document your design
This last non-functional requirement requires you to document your design decisions and implementation changes. The aim of this is to communicate what you have done to a reader. Your document should look professional, have clear sections, and be written clearly.
Complete these steps:
1. Create adocument that will present your design.
2. Add a section that presents a complete design class diagram of the final design.
a. Highlight every aspect (classes, relationships, etc) that you have added to the design.
3. For each major Functional Requirement (FR1, FR2, FR3, FR4), add a named section that presentshow you addressed that requirement from a design and
implementation perspective:
a. Name the design pattern you chose.
b. Explain why you applied that particular design pattern (one paragraph).
c. Show a class diagram that highlights the specific classes in the system and relationships that address that design pattern.
d. Explain, step by step, how your design works. A numbered list may work best here. Reference your diagrams as necessary.
e. Reference the git commits by commit ID where you addressed this aspect of the design.
Note: an example of a reasonable design description for this section is presented in Appendix 2.
General requirements
This section presents general requirements for the assignment that must be adhered to.
Design constraints
The following design constraints must be adhered to in your code:
DC1. The Visual Studio 2022 CMake project you submit must compile and run.
DC2. C++ standard library collections must be used for storing collections of objects.
DC3. You must use dynamic memory where appropriate (e.g. new and delete or smart pointers).
DC4. Dynamically allocated memory must be cleaned up correctly.
DC5. Function parameters must use references appropriately.
DC6. Collections of pointers must be cleaned up correctly.
DC7. Class member functions should be declared const unless they cannot be.
DC8. Initialiser lists must be used for constructors where initialisation is required.
DC9. The code style guide available on the course website must be followed with the following exception: you can decide whether to place opening braces on the
statement line or the following line.
DC10. Your system must not use external libraries (e.g. BOOST).
Assignment and submission requirements
The assignment has the following general requirements. Failing to address any of these requirements will result in deducted marks.
1. The assignment must be submitted via LearnOnline.
2. You must submit three files: 1) a zipped Git repository (.ZIP), 2) a functioning executable (.EXE), and 3) the system design document (.PDF)
3. These files must be submitted as three separate files to LearnOnline.
4. The GIT repository must:
a. Include the full GIT history;
b. Include the CMakeLists.txt file and all other project files EXCEPT the .vs and out folders
c. It must build and run from Visual Studio 2022 on Windows.
d. The repository must be compressed using the common ZIP compression.
5. The functioning executable must:
a. Run from command line under Windows 10 or higher.
6. The system design document must:
a. Be submitted as PDF format.
b. Note: it will also exist as Word document or similar in your repository.
Workplan
The following workplan is suggestion. First, do not wait until the due date to start the assignment; under the “GIT” marking criteria, we are expecting to see consistent commits each week. You can progressively build the system as your understanding of design patterns develops.
When creating the Git repository, make sure to use the .gitignore file provided on the course’s LearnOnline website. If you forget it, your assignment will be too large to upload on LearnOnline!
The functional requirements have been presented in a logical order for implementation. As you implement each requirement, document the design and implementation. When you have finished, review your design document and update to correctly reflect your code.
Constantly commit to GIT. Use it as a safety net so that you can always revert to a working version of your project.
Marking Scheme
Criteria |
Mark |
|
Design document (5%) Overall presentation – Presentation, consistent visual style, spelling and grammar, diagrams legibility. Design (55%) |
5% |
|
Each of these criteria are marked from the design document. They will be marked on 1) completeness of diagram and syntax, 2) correctly selected and applied design pattern, 3) clarity of explanation, 4) referenced git commits, and 5) correctly reflecting the code. FR1: Calculate the patient alert levels for their primary disease 15% FR2: Calculate the patient alert levels for all their diseases 10% FR3: Load patients from file 15% FR4: Alert the hospitals and GPs 15% Functionality and implementation (20%) Functionality – The program functions as expected. Patient alert 20% levels are correctly reported. Patient data is correctly loaded. Implementation - The design patterns are implemented correctly. Non-functional requirements (20%) GIT – GIT has been used. Optimal marks are rewarded for 1) at least 10% one commit each in Week 11 and Week 12 and 2) at least one commit per functionality in the system. Commit messages should use the imperative mood. Design constraints – The design constraints have been addressed in 10% the code implementation. |
• You will be required to explain your design and code during week 13. Not being able to explain any of these elements can result in losing marks.
Extensions
Late submissions will not be accepted for this course unless an extension has been approved by the course coordinator. Late submissions that have not been approved will receive a
mark of zero. Refer to the course outline for further information regarding extensions.