assignment
Learning Outcomes
In this assignment you will demonstrate your understanding of arrays, strings, functions, and the typedef facility. You must not make any use of malloc() (Chapter 10) or fifile operations (Chapter 11) in this project, and should probably also stay away from struct types too (Chapter 8).
Files of Number
Vast quantities of scientifific and engineering data are stored in comma separated values-format (CSV) fifiles. In such a fifile the fifirst line usually describes the columns, and then all the other rows contain numeric data. For example, the fifirst few lines of the test fifile data0.txt consist of:
year,month,day,location,mintemp,maxtemp
2020,8,28,18,6.7,12.9
2020,8,28,22,12.7,19.1
2020,8,29,18,7.6,15.3
and show three rows of data for temperatures in August 2020, with a code for a location (maybe “18” is Melbourne and “22” is Sydney), a minimum temperature, and a maximum temperature recorded.
Your task in this assignment is to develop a kind of “CSV tabulator”. You will use a two dimensional C array to store a matrix of numbers, and will write functions that carry out operations on the stored data, including generating reports, graphing it, and sorting it. All of the numbers in all of the input data should be treated as being double, even if they do not include decimal points; and two numbers should be regarded as being equal if they differ by less 10!6. Apart from the fifirst row, all data will be strictly numeric.
Before doing anything else, you should copy the skeleton program ass1-skel.c and sample data fifile data0.txt from the FAQ page1, and spend an hour (or two!) to read through the code, understand how it fifits together, and check that you can compile it via either grok or a terminal shell and gcc. Note that if you plan to use grok, you will also need to create test fifiles as part of your project, and will need to learn how to execute programs in grok via the “terminal” interface that it provides. In other words, now might be a good time to step away from the comfortable environment provided by grok and commit to genuine “shell”-mode C programming on your computer.
The skeleton program provides a main program, and two further functions that are somewhat tedious to implement. In particular, the CSV data fifile is read and processed into internal format (the type csv t) by the function get csv data(); and the function get command() is provided, together with a controlling loop in the main program, to help you with the interactive input. You do not need to understand the way in get csv data() works, but should be able to by the end of the semester (therelevant techniques are described in Chapter 11). The function get command() should make sense
to you by the end of the Week 6 lecture videos. You are to use these two functions and the main() function without making any modififications to them.
Once you have ass1-skel.c compiled, try this sequence:

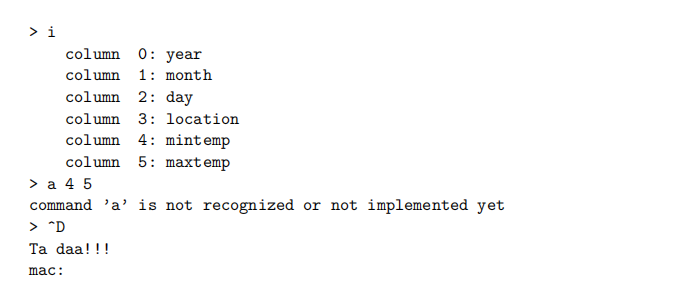
Note that data0.txt is provided as an argument to the program. That fifile is opened and read as soon as the program commences (magic!) and then the fifirst “>” is printed by the program, as a prompt to say “ready for instructions”.
The program maintains the numeric CSV data internally in a two-dimensional array D[][] of type csv t and buddy variables dr and dc (the number of active rows and columns respectively), with the row header strings stored in a separate array H[] of type head t (and which also uses dc as buddy variable). Those are the primary data structures that you need to manipulate in the following stages.

Stage 1 – Averaging and Displaying (12/20 marks)
Ok, now time for you to add some new commands, starting with ’a’. Write and incorporate a function do analyze() that takes the standard set of arguments (see do index()) and for each column that is listed in ccols[] (buddy variable nccols), provides some overall stats about that column of data:
Stage 3 – Plotting (20/20 marks)
General Tips...
You will probably fifind it helpful to include a DEBUG mode in your program that prints out interme-diate data and variable values. Use #if (DEBUG) and #endif around such blocks of code, and then #define DEBUG 1 or #define DEBUG 0 at the top. Turn off the debug mode when making your fifinal submission, but leave the debug code in place. The FAQ page has more information about this.
2020-09-23