CPEN 231L Lab #8 –Fixed Point Arithmetic
Hello, dear friend, you can consult us at any time if you have any questions, add WeChat: daixieit
CPEN 231L
Lab #8 –Fixed Point Arithmetic
1. Write an assembly program for Cortex-M4 to compute the value of Z using fixed point integer arithmetic. The constants in the formula are in decimal representation:
Z=24.513*Num-415.285
2. The BCD value of Num is read from the keypad (using the subroutines from Lab7). The program will display a prompt message and then read the value of Num from the keypad (2 BCD digits). This value of Num shall be converted into its binary equivalent number (as in Lab2) and stored in the two middle bytes of register R1 prior to computing Z.
3. The result Z shall be stored in 3 bytes (24-bit variable with hexadecimal point placed between middle and low byte). The fractional portion of each constant shown in the formula for Z shall be approximated with 12 bits. Since there is only 1 byte to represent the fractional portion of Z, the factional portion is rounded off to an 8-bit number (or 2 hexadecimal digits)
Z (3 bytes) |
|||||
Z+2 |
Z+1 |
Z |
|||
|
|
|
|
|
|
4. The constant (415.285)10 = (19F.483)16 should be defined as constant of 3 bytes in length as
Const (415.285) |
|||||
Const+2 |
Const+1 |
Const |
|||
0 |
1 |
9 |
F |
4 |
8 |
5. Write a subroutine Bin2ASCII that converts an 8-bit binary number into two ASCII characters corresponding to its hex value. The binary value should be passed through R1. After the conversion, the ASCII codes of the LS and MS hex character should be returned in the lower two bytes of R2 (notice that to get the ASCII code for a decimal digit character, add #0x30; and for a letter character, add #0x37).
6. Write a subroutine to display the hexadecimal value of Z on LCD using the routine developed in previous steps. For example, if Num = (16)10, Z = (FFE8.ED)16, the contents will be displayed as
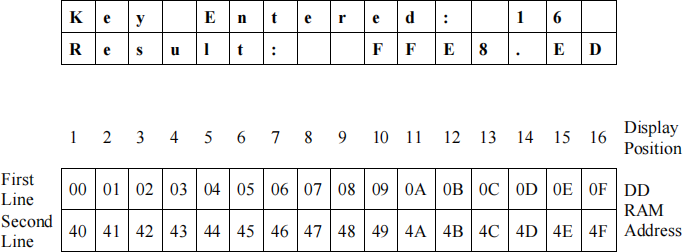
In preparation for the lab
7. Study carefully the flowchart for the program below and write subroutines Read_Number, Compute_Z and Display_Z.
8. Select a couple of values for Num(one yielding a positive result and the other a negative result). For each of these values compute the hexadecimal value of Z.
During the lab
9. Create a new project, namely Lab8. Add the appropriate block ID.
10. Create a new assembler source file (e.g., LCD.s) and copy the LCD-related code to the file. Similarly, create another new assembler source file (e.g., keypad.s) and copy the keypad- related code to the file. In each of these two files, export all the subroutines (e.g., Init_LCD, Display_Msg) and global variables (e.g., Key_ASCII) that will be used by each of the other file and the current lab. For example,
THUMB
AREA LCD_Code, CODE, READONLY, ALIGN=2
EXPORT Key_ASCII
EXPORT Init_LCD_Ports
EXPORT Init_LCD
……
To use these subroutines and global variables in another source file, you need to import them. For example,
AREA MyCode, CODE, READONLY, ALIGN=2
EXPORT main
IMPORT Init_LCD_Ports
IMPORT Init_LCD
……
11. When the program runs properly, change the program into a cyclic operation allowing the values to be computed continuously. When the cyclic operation is tested, let the instructor test and check off the program.
12. Generate the “ .lst” file of this lab, and print it out. Submit it along with the prelab assignment, the test data, and the results obtained if any. The quality of design and documentation will be graded.
Note:
Here is the explanation of how to implement Z:
The value of Z will be displayed on LCD as
2024-04-04