CS170 – Computer Applications for Business Spring 2024 • Assignment 7
Hello, dear friend, you can consult us at any time if you have any questions, add WeChat: daixieit
CS170 – Computer Applications for Business
Spring 2024 • Assignment 7
Event Driven Programming
Due Date: |
Before 11:59 p.m. on Friday, March 22nd, 2024 |
Accept Until: |
Before 11:59 p.m. on Friday, March 29th, 2024 |
Evaluation: |
25 points |
Submit to Canvas: |
Assignment7.html file |
Related Materials: |
JavaScript Reference Guide |
Need help? |
TA office hours and Lab Support Schedule posted in Canvas |
YOU ARE ON YOUR HONOR TO COMPLETE THIS ASSIGNMENT WITHOUT ASSISTANCE. You may use your text and resources available to you through Canvas. You MAY NOT ask any person for assistance, give assistance to anyone, or use any online tutoring service. |
|
Use only the coding syntax included in the JavaScript Reference Guide, the section “Additional Information” of this document and the syntax for input elements (text boxes, radio buttons, buttons) in chapter 18 of the textbook.. The use of other coding syntax will result in your work being marked for further review due to possible unauthorized assistance. |
Learning Objectives:
This assignment is designed to practice:
1. Creating an Event Driven JavaScript program.
2. Using Input Elements such as text boxes, buttons, and radio buttons in an event driven program
3. Implement JavaScript code which will respond to user events.
4. Use of variables: declaration, initialization, modification
5. Use of comments.
To earn credit for this assignment:
1. You will create a JavaScript program – embedded on an HTML file - according to the requirements listed on the next pages.
2. Upload and submit a single html file (.html extension) containing the code required.
For your assignment to be graded, please include the following statement: “ On my honor, I have neither received nor given any unauthorized assistance on this assignment.”
PROBLEM DESCRIPTION
A GUI (Graphical User Interface) is required to process pets spa services and produce accurate results. Radio buttons and text boxes will be used for input and output purposes. A button will be used for processing data. Another button will be employed to clear the text boxes and radio buttons.
Create a webpage to implement the interface required using the JavaScript programming language. The illustration below displays the format of the GUI screen required. An HTML template is provided on Canvas to be used as a starting point to create the screen required.
Note: Choose a background color and a text color of your preference.
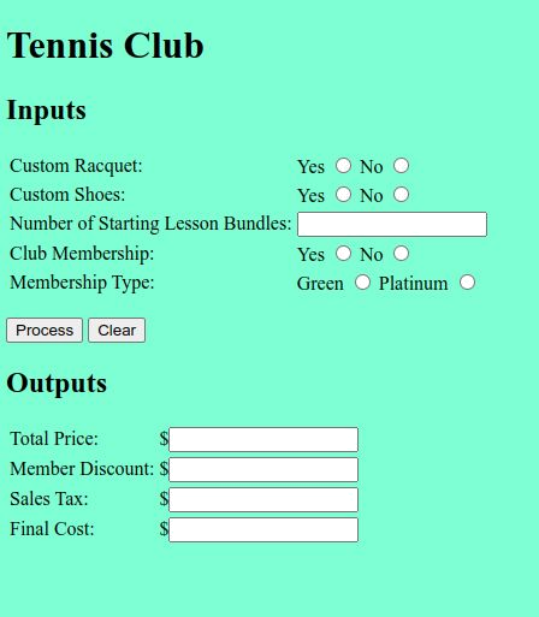
The rules about the logic required are the same as those for the initial version and are listed below:
The Shady Brook Tennis club recently experienced a boom in interest following one of their players qualifying for Wimbledon. To take advantage of the increase foot traffic, the tennis club is
expanding their offerings, both in their tennis shop and their lessons, for new players.
. The equipment starter package:
◦ Custom racket fitting $150
◦ Custom shoe fitting $100
. The lessons starter package:
◦ Lessons can be purchased in bundles of 5 sessions for $200
◦ Purchases of 5 (or more) bundles will receive a 10% discount
. At the end of the purchase, two levels of club memberships will be offered, Green or Plat- inum. Customers can choose either one (or none) of the two.
◦ Green level membership cost $50 and provides an additional 10% discount on equip- ment
◦ Platinum level membership costs $200 and provides a 20% discount on all purchases(in- cluding the membership cost)
. Inputs:
. EquipmentRacket: Yes or No
. EquipmentShoes: Yes or No
. NumLessonBundles: Integer
. ClubMembership: Yes or No
. MembershipType: Green or Platinum
.
. Outputs:
. Total price (pre-tax).
. Member Discount.
. Sales Tax amount to be added to the bill (7% rounded to two decimals).
. Final Cost of the bill (including the tax).
Processing:
. Process button:
◦ Notice that the Process button does not need to “read” the radio buttons since the radio buttons take care of loading the appropriate value to the variables
included in their onclick properties.
◦ It will process the inputs gathered by the radio buttons to perform the appropriate calculations and generate the required results.
▪ The code for the Process button should not include the prompt or alert
functions
◦ It will place the required results on the appropriate output boxes.
▪ Example of the syntax for writing to a text box: . myTextBoxID.value = myVariable;
◦ It writes the content of the variable myVariable to the text box whose ID is myTextBoxID.
. Clear button:
◦ It will reset all variables
▪ The numeric variables should be set 0
▪ The string (text) variables should be set to the empty string.
◦ All the text boxes should be loaded (written to) with the empty string to clear them of any content.
◦ It will also clear all the radio buttons (see examples of clearing radio buttons in the Additional Information section).
SAMPLE TRACES THROUGH THE CORRECT ALGORITHM
|
Sample 1 |
Sample 2 |
Sample 3 |
Inputs: |
|
|
|
Custom Racket: |
No |
Yes |
Yes |
Custom Shoes: |
Yes |
No |
Yes |
Starter Lesson Bundles: |
0 |
3 |
5 |
Membership Club: |
No |
Yes |
Yes |
Membership Type: |
|
Green |
Platinum |
Outputs: |
|
|
|
Total Price |
$100.00 |
$800.00 |
$1350.0 0 |
Member Discount |
$0.00 |
$15.00 |
$270.00 |
Sales Tax |
$7.00 |
$54.95 |
$75.60 |
Final Cost |
$107.00 |
$839.95 |
$1155.6 0 |
|
|
|
|
Additional Information:
. Be sure to include a comment with your name and section in the <head> part of your HTML code program.
. To simplify the comparison of text data entered by the user, it is often easier to convert this text to upper case using the notation below.
Syntax example:
textVariable = textVariable.toUpperCase();
(See https://www.w3schools.com/jsref/jsref_toUpperCase.asp for additional example.)
. Syntax example to round a number to 2 decimals. Just add .toFixed(2) to the variable name containing the number.
someNumber.toFixed(2)
. Syntax example to use process a number that was originally captured via a text box:
. numberWins = Number(numberWins);
. Syntax example to read input from a text box:
. x = someBoxId.value;
Where x is a variable and someBoxId the Id of a text box used for input
. Syntax example to write output to a text box:
. someOtherBoxId.value = y;
Where y is a variable and someOtherBoxId the Id of a text box used to display output
. Examples related to radio buttons (these are examples only, the name, id and onclick content should be related to your code):
. Using radio buttons:
<input type="radio" name="size” id="large" onclick='sizeData="L"'>
<input type="radio" name="size” id="small" onclick='sizeData="S"'>
. In the example above, the two associated radio buttons, load the appropriate value
to the variable sizeData based on the user’sselection.
. Clearing radio buttons:
. The radio buttons in the previous example may be cleared by using the following syntax in a program:
. large.checked = false;
. small.checked = false;
. Notice that the IDs of the radio buttons are used when clearing a radio button.
Point Values
Student name inserted as comment in the head section |
1 |
Screen format with radio buttons |
4 |
Screen format with text boxes |
2 |
Screen format with buttons |
1 |
Variable declarations |
2 |
Variable initializations |
2 |
Process Button with appropriate code for reading input |
2 |
Process Button with appropriate code for writing output |
2 |
Clear Button clears text boxes and radio buttons |
1 |
Clear Button resets variables |
1 |
Program execution (produces results) |
2 |
Program execution (correct results) |
5 |
References:
. Recitation sessions
. Lectures
. Fluency textbook Chapters 17, 18
. HTML color names: https://www.w3schools.com/colors/colors_names.asp
2024-03-11
Event Driven Programming