ITS63304 OBJECT ORIENTED PROGRAMMING
Hello, dear friend, you can consult us at any time if you have any questions, add WeChat: daixieit
FINAL EXAMINATION
AUGUST 2023 SEMESTER
MODULE NAME : OBJECT ORIENTED PROGRAMMING MODULE CODE : ITS63304 EXAM DURATION : 2 HOURS This paper consists of six (6) printed pages, inclusive of this page. |
MLO |
Section(s)/ Question(s) |
Marks |
MLO1 |
Questions 1: a, b,c |
/ 15 |
MLO2 |
Questions 2: a,b,c |
/ 15 |
|
TOTAL |
/ 30 |
MARKING SCHEME
Question 1 (15 marks)
a. Describe the following Object-Oriented concepts as they pertain to Java Programming. For each concept, provide a brief description and its significance. (5 marks)
i. Class:
. Description: A class is a blueprint for creating objects in Java, defining their structure and behavior.
. Significance: Classes enable code organization, reusability, and the modeling of entities in a program.
ii. Object:
. Description: An object is an instance of a class, representing a tangible entity with attributes and methods.
. Significance: Objects are the building blocks of Java programs, allowing manipulation of data and behavior.
iii. Inheritance:
. Description: Inheritance allows a subclass to inherit properties and behaviors from a superclass, promoting code reuse.
. Significance: Inheritance fosters hierarchy and relationship between classes, reducing code duplication.
iv. Polymorphism:
. Description: Polymorphism enables objects of different classes to be treated as instances of a common superclass.
. Significance: It provides flexibility by allowing objects to respond differently to the same method call, simplifying code design.
v. Encapsulation:
. Description: Encapsulation bundles data and methods into a class, restricting direct access to data.
. Significance: It enhances data protection, code maintainability, and controls how data is accessed and modified.
b. Define and explain syntax and semantics compilation errors presented. How do syntax
errors differ from semantic errors in terms of identification and resolution? (6 marks)
Syntax Errors – These are errors where the code doesn't adhere to the rules of the programming language. They are usually straightforward to identify because compilers provide clear feedback about them. For example, missing semicolons or unmatched parentheses. Due to the clear feedback, they are often considered the easiest type of errors to fix. [2 marks]
Semantic Errors - These are errors where the code compiles correctly but doesn't behave as intended. This can be due to incorrect logic or misuse of a programming construct. They can be harder to identify because the compiler won't flag them; the program just won't work as expected. [2 marks]
When contrasting, syntax errors are usually more straightforward to identify and fix because of clear compiler feedback, whereas semantic errors require a deeper understanding of the program's intended behavior and logic. [2 marks]
c. Given a piece of code, evaluate its robustness by identifying potential syntax errors. How would these errors impact functionality and performance of the program if left unresolved?
int a = 5
x = ( 3 + 5;
y = 3 + * 5;
(4 marks)
Error 1 − Missing semicolon • int a = 5 // semicolon is missing [0.5 mark]
Errors 2 in expression • x = ( 3 + 5; // missing closing parenthesis [0.5 mark] Error 3 • y = 3 + * 5;// missing argument between ‘+‘ // and '* '[1 mark]
Question 2 (15 marks)
a. Write a java program that processes a name entered by the user. The requirements:
i. Prompt the user to enter their full name (first and last name)
ii. Extract the first and last name from the input. You can assume that the first and last
names are separated by a space and that the user enters a valid name format.
iii. Use methods of the String class to manipulate the name as specified: Display the last name followed by a comma, then the first initial of the first name. For example, for the input “John Doe”, the output should be “Doe, J” .
iv. Hints: Scanner, charAt(), and toUpperCase() methods of the String class useful for this task.
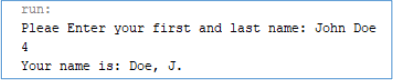
(5 marks)
import java.util.Scanner;
public class Processaname {
public static void main(String[] args) {
Scanner count = new Scanner(System.in);
System.out.print("Pleae Enter your first and last name: ");
String name = count.nextLine();
int space = name.indexOf(" ");
System.out.println(space);
String first = name.substring(0, space);
String last = name.substring(space + 1, name.length());
String firstInitial = first.substring(0, 1);
name = last + ", " + firstInitial + ".";
System.out.println("Your name is: " + name);
}
}
i. Prompt the user to enter their full name (first and last name) (1 mark)
ii. Extract the first and last name from the input. You can assume that the first and last names are separated by a space and that the user enters a valid name format. (1 mark)
iii. Use methods of the String class to manipulate the name as specified: Display the last name followed by a comma, then the first initial of the first name. For example, for the input “John Doe”, the output should be “Doe, J”. (3 marks)
iv. Hints: Scanner, charAt(), and toUpperCase() methods of the String class useful for this task.
b. Case Study: Refinement of Stock Order System (10 marks)
You're given an initial framework for a Stock Order System tailored to a local retailer's needs. The present system supports functionalities like adding stock items, ordering, inspecting available stock, and reviewing past orders.
Objective: Your task is to augment and fine-tune these features, ensuring the system offers optimized stock management capabilities.
i. Enhance Stock Management
a. Implement a feature to update the quantity of an existing stock item
b. Ensure that when adding a new stock item, it checks if the item already exists. If it does, update the quantity instead of adding a new entry.
ii. Functionality
a. Implement a search feature that allows users to search for a stock item by name and view its quantity.
b. The search should be case-insensitive.
iii. Hints:
a. Search the ‘orders’ ArrayList to find the order to cancel.
b. Update the quantity of the related stock item when an order is canceled.
c. Use Java Streams or a loop to filter and find a stock item by name in the ‘stockItems’ ArrayList.
d. Utilize the ‘equalsIgnoreCase’ method of the ‘String’ class for case- insensitive comparison.
import java.util.ArrayList;
import java.util.Scanner;
class StockItem {
private String itemName;
private int quantity;
public StockItem(String itemName, int quantity) {
this.itemName = itemName;
this.quantity = quantity;
}
public String getItemName() {
return itemName;
}
public int getQuantity() {
return quantity;
}
public void setQuantity(int quantity) {
this.quantity = quantity;
}
}
class Order {
private String itemName;
private int orderedQuantity;
public Order(String itemName, int orderedQuantity) {
this.itemName = itemName;
this.orderedQuantity = orderedQuantity;
}
public String getItemName() {
return itemName;
}
public int getOrderedQuantity() {
return orderedQuantity;
}
}
public class StockOrderSystem {
private static ArrayList<StockItem> stockItems = new ArrayList<>();
private static ArrayList<Order> orders = new ArrayList<>();
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("Welcome to the Stock Order System!");
while (true) {
System.out.println("1. Add Stock Item");
System.out.println("2. Place Order");
System.out.println("3. View Available Stock");
System.out.println("4. View Orders");
System.out.println("5. Exit");
System.out.print("Choose an option: ");
int choice = scanner.nextInt();
scanner.nextLine(); // consume newline
switch (choice) {
case 1:
System.out.print("Enter stock item name: ");
String itemName = scanner.nextLine();
System.out.print("Enter stock quantity: ");
int quantity = scanner.nextInt();
stockItems.add(new StockItem(itemName, quantity));
System.out.println("Stock item added!");
break;
case 2:
System.out.print("Enter stock item name to order: ");
String orderItemName = scanner.nextLine();
System.out.print("Enter quantity to order: ");
int orderQuantity = scanner.nextInt();
StockItem item = stockItems.stream().filter(s ->
s.getItemName().equalsIgnoreCase(orderItemName)).findFirst().orElse(null); if (item == null) {
System.out.println("Item not found in stock.");
} else if (item.getQuantity() >= orderQuantity) {
item.setQuantity(item.getQuantity() - orderQuantity);
orders.add(new Order(orderItemName, orderQuantity));
System.out.println("Order placed!");
} else {
System.out.println("Insufficient stock.");
}
break;
case 3:
System.out.println("Available Stock:");
stockItems.forEach(stock -> System.out.println(stock.getItemName() + " - " + stock.getQuantity()));
break;
case 4:
System.out.println("Placed Orders:");
orders.forEach(order -> System.out.println(order.getItemName() + " - " + order.getOrderedQuantity()));
break;
case 5:
System.out.println("Goodbye!");
return;
default:
System.out.println("Invalid choice. Please try again.");
}
}
}
}
Marking scheme:
i. Declaration correctly defined [2 marks]
ii. Functionality listed with the requirements [5 marks]
iii. Uses the hints with all the availability [1]
iv. Running program [2 marks]
2024-02-05