COMP2005J Object Oriented Programming
Hello, dear friend, you can consult us at any time if you have any questions, add WeChat: daixieit
COMP2005J Object Oriented Programming
Course project description
You need to implement a Car rental system in java using object-oriented programming. You need to implement your code by using at least 3 of the main pillars of Object oriented programming (Encapsulation, Inheritance, Polymorphism). This means that you need to use Interfaces, Nested Classes, Class hierarchy and other features related to these concepts as necessary.
* Encapsulation: By creating classes for Car, Reservation, User, and Admin and wrapping data and methods that operate on the data into a single unit.
* Inheritance: By using subclasses for different Car Categories types ( SUV, SEDAN, Sports) which will inherit properties from a parent Car class.
* Polymorphism: By utilising interface methods that can be implemented differently for user and admin, allowing different behaviours for the two user types.
System Entry:
Upon starting the application, users are greeted with a main menu (Command line Interface):
● User: This option directs the user to the user panel.
● Admin: This option directs the user to the admin panel.
● Exit: This option allows the user to exit the application.
User Panel:
In the user panel, users can:
● See Available Cars for Rent: Users can view the list of cars available for rent. If there are no cars available, a message indicating the unavailability is displayed.
● Rent a Car: Users can choose to rent a car.
● See Your Reservations: Users can view their car rental reservations.
● Return a Car: Users can return a rented car.
● Exit: Users can go back to the main menu.
Admin Panel:
In the admin panel, administrators can:
● Add a Car: Administrators can add a new car to the inventory.
● See All Cars: Administrators can view the entire list of cars in the inventory.
● Delete a Car: Administrators can remove a car from the inventory.
● Exit: Administrators can go back to the main menu.
Car Configurations:
* You do not need to use a database to store the inventory. You only need to keep track of the inventory during the runtime of the code and the inventory should reset when you end the execution.
● Car Categories and Rates:
● SEDAN: Hourly rate RMB90, Daily rate RMB350, Weekly rate RMB900
● SUV: Hourly rate RMB120, Daily rate RMB450, Weekly rate RMB1100
● SPORTS: Hourly rate RMB150, Daily rate RMB550, Weekly rate
RMB1400
Each car has attributes:
● Category (SEDAN, SUV, SPORTS)
● Model ("BMW", "Porsche", "Audi")
● Quantity
Model Rate Adjustments:
● Rates are subject to adjustments based on the car's model:
● BMW: 20% of the total bill.
● Porsche: 30% of the total bill
● Audi: 10% of the total bill
should be added to the bill, if the user chooses the particular model.
Tasks:
1. Implement fully functional User Panel as stated above. Upon renting a car, the user should be able to see the final bill. Also, the user should see the billed amount while seeing the reservation. After renting or returning the car, the available cars to rent should be updated. For instance, see the following:
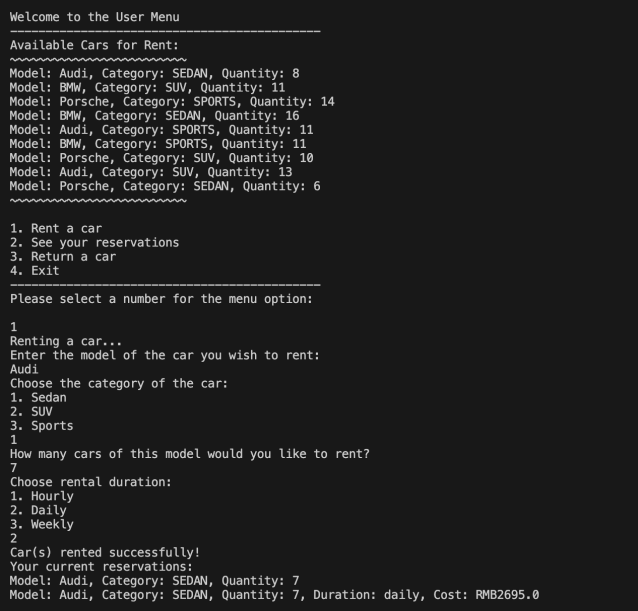
Available cars for rent should be updated as the following:
2. Implement fully-functional Admin Panel as stated above. Admin should be able to add/delete a car from the inventory. It should impact the available cars to rent.
See the current Inventory after adding new car:
See the available cars to rent in the user panel:
The available cars to rent for Audi, Sedan becomes 13. Because the user rented 7 cars of the same model before. So, the inventory has 20 Audi, Sedan, but only 13 of them are available for rent.
Test Scenarios:
You should record your screen when running the following tests and submit a video inside the zip file. The video duration should be less than two minutes, and you should explain by talking to the microphone during the recording.
● The Admin adds 10 BMWs (1 Sport, 5 Sedan, 4 SUVs).
● The customer tries to rent 4 SUVs of the model "BMW" for one day and will be billed considering both the SUV daily rate and the BMW model adjustment.
● The customer tries to rent 2 SUVs and receives an error: “We do not have enough cars” .
● The customer returns 1 BMW SUV and receives a promt saying (Returned Sussecfully!).
● The Customer checks the reservations and now it should only be able to see 3 BMW SUVs in the reservation list.
Bonus Point (10% Extra):
To get the extra 10% the rental system should implement a Graphical User Interface (GUI) to allow the users to interact with it. Here is a Tutorial and a video guide on creating GUIs in IntelliJ Swing.
Grade component
Project submission (40% of full grade).
Submission deadline
The submission deadline is Thursday, November 30, 2023, at 23:59 hours (Beijing time).
Submission guidelines
Your submission should include:
● Source-code of your implementation application, implemented in java. You should submit your code as a project and not as a single file. For this you should export your project as a zip file from the IDE (See how to do it on IntelliJ).
● The screen recording of test scenarios (less than 2 minutes). If you have a GUI you should also record the test scenarios using that.
● A detailed configuration report (in PDF format) describing your implementation of the course project. This report should describe in detail how you have implemented the project, and the various challenges that you faced during the same. This manual should describe the step-by-step procedure of developing your code maximum 7 pages including cover and references.
Please upload a single zipped folder (in .zip format) containing all these files arranged in a systematic manner.
The naming convention for each of the projects is:
ucd connect id_pM.zip
where ucd connect id is your UCD Connect ID, M is the project number, and p and zip are in lower-case characters.
For example, a student John Smith with the UCD Connect ID 12345678 would submit the first project as 12345678_p1.zip.
Grading scheme
The penalties for late submission are as follows:
● Less than 15 minutes past the deadline - no penalty.
● Greater than 15 minutes and less than 2 hours past the deadline - loss of 25% of the final mark.
● Greater than 2 hours past the deadline - loss of 50% of the final mark
The submission will be graded based on the following criteria:
● Correctness: The software should perform as described in the final report.
● Robustness: The software should operate reliably and be not subject to frequent abnormal terminations.
● Report Quality: The associated configuration report should be organised and well-structured and.
● Use of Object Oriented Programming Concepts (Encapsulation, Inheritance, Polymorphism).
● Video presentation: You should explain steps of the test during the recording.
If you have any queries about the Project details:
1- Create a new topic in the Brightspace Discussion Forum under the Project Q&A. 2- In case of personal circumstances Contact your TA ([email protected])
3- Do not leave your questions for the days closer to the deadline as we might not be able to answer all the questions in a short time.
2023-11-29