MCD4290 T2-2023 Assignment 1
Hello, dear friend, you can consult us at any time if you have any questions, add WeChat: daixieit
MCD4290 T2-2023
Assignment 1
Due Week 8 Friday 11:55 PM
Preface
Assignment 1 is an individual coding portfolio assignment
Warning
You must read the entire assignment and make sure you understand the features and functionality of the entire web app before starting.
Submission
You will need to submit the assignment as a ZIP file to Moodle under the submission link provided in the section 'Assignment 1'. See 'Submission and Late Penalties' for more information. The section 'Marks and Scaling Factors' has information about the marking and factors applied to your assignment.
As part of this assignment, you will be required to complete a reflection activity. This unlocks once the submission closes and you will have seven days after the assignment deadline to complete it. Non-completion of the reflection activity will result in your assignment marks being capped at 50% of the available marks.
Your work will run through a plagiarism and collusion detection system from Stanford University.
TL;DR: This is an individual assignment, so you must work on it by yourself. If you have any questions, you should ask them on the forums and we will help you.
See the 'plagiarism and collusion warning' below. If you're unsure what any of it means, please ask your campus lecturer.
Resources
JavaScript Reference:MDN [link]
HTML Reference:MDN [link]
MDL Reference:getmdl.io [link]
Plagiarism and Collusion Warning
We will use MOSS to detect plagiarism and collusion in the assignment.
You are not allowed to search online for answers or for help on assignment work (anything that is assessed), except for links provided in the question and in the instruction 'resources'.
Reproduction of this assignment online is an academic misconduct offense.
You are also not allowed to ask anyone except unit staff for help as it is an individual assignment, including discussing the question logic, etc.
You are allowed to seek help from the unit staff via the unit forums or during helpdesk.
Plagiarism and collusion are serious academic offenses at Monash University. Students must not share their work with others. Students should consult the policy linked below for more information.
https://www.monash.edu/students/academic/policies/academic-integrity
https://www.monash.edu/engineering/current-students/enrolment-and-re-enrolment/course- information/assessment-and-examinations/academic-integrity-and-plagiarism
Students involved in collusion or plagiarism will be subject to disciplinary penalties, which can include:
● The work not being assessed
● A zero grade for the unit
● Suspension from the University
● Exclusion from the University
Special Consideration
Special consideration applications are to be made online via your student portal on Moodle.
Marks and Scaling Factors
Marking
Your assignment will be assigned marks per task.
A Marking Rubric is available for your reference in the Assignment 1 section on Moodle.
Assignment 1 is worth 8% of your unit marks.
There are penalties that will apply to the assignment (deducted once for the entire assignment)
● Not having appropriate code Indentation and Code Brackets (per the style guide)
● Not having appropriate variable and function naming (per the style guide)
● Not having appropriate variable and function scoping (smallest possible)
● Not having appropriate function header documentation (name, purpose of function, parameter and return value description)
● Use of magic numbers (per the style guide)
● Not having appropriate file header documentation (name, purpose/description of file)
● You should also start each JavaScript file with "use strict"; to use strict mode
checking. You should also adhere to other good programming practices that you will learn through working on the assignment, and through examples from your demonstrator. There is a coding standards document found on Moodle under the ‘resources’ heading in the Assignment 1 section.
Other Scaling Factors
Upon submission, you must also complete the reflection activity. Non-completion of this activity will result in your marks for the assignment capped at half the total marks.
You can find the reflection activity under the 'Assignment 1' section, directly below your submission link for the assignment.
This reflection activity will open when the Assignment submission closes, and you will have seven days to complete this from the Assignment deadline.
Marking Criteria
Your work will be assessed based on the work submitted to Moodle.
Criteria:
● Whether the functionality of the code satisfies the specifications
● quality of code, including structure and whether it meets coding standards
Feedback
Feedback will be returned via Moodle, within 2 weeks after submission.
Submission and Late Penalties
Submission
Your final submission must follow these requirements:
A single zip file named as XXXXXXXX-A1.zip, where XXXXXXXX is replaced by your 8 digit student ID (i.e. 12345678).
This must be a ZIP file and not other types of compressed folder.
Instructions on how to create a zip file
The zip file should contain the prescribed folder structure and files based on the information provided in the Tasks section.
Reflection Activity for Assignments
Instructions
Non-completion of this task will result in the marks for the assignment being capped at 50% of its value. If your submission does not properly reflect (i.e. a serious attempt), your marks may be capped at 50% anyway. We strongly suggest that you do not leave this to the last minute, and if unsure if your submission construes a proper reflection, to check with your
demonstrator first before submitting.
You will have seven days from the assignment deadline to complete this reflection.
Task
Write a 100-300-word reflection on the following:
For the assignment,
1. Describe one question or section that you found challenging.
2. In attempting the section or question identified above, discuss what did you do to overcome the challenge, and then
3. Briefly describe what did you learn from it.
Late Submission Penalty
Unless an extension or special consideration has been granted, students who submit an
assessment task after the due date will receive a late-penalty of 10% of the available marks in that task per calendar day. Assessment submitted more than 7 calendar days after the
due date will receive a mark of zero (0) for that assessment task. Students may not receive feedback on any assessment that receives a mark of zero due to late-submission penalty.
There is a 30 minute leeway on the main assignment deadline, unless an extension, special consideration has been granted, or if the assessment was submitted late; in which case the 30 minutes do not apply.
I need help
The unit staff will be available should you need help.
You can also ask questions about the assignment on the Forums. This is the preferred venue for assignment clarification-type questions.
Tasks
Case
You work with a software development company and the company is interested in building their own Project progress tracking web app. You have been commissioned to take over the development of the initial prototype. The application design has been worked out by your team leader, and the documentation is provided as follows:
You will need to download the provided skeleton files in the Assignment 1 section on Moodle.
This is a project progress tracking system that your team can use to keep track of their progress by keeping a card for each task with status information. A task can have one of these statuses: to do, in progress or completed. A task can be locked once it's completed and then becomes non-editable.
You will note that each file has a placeholder or TODO comment for where you should write the code for that specific task.
Setup:
You are provided with six files:
● Two HTML files
○ index.html => 'main page'
○ view.html => 'view page'
● Three JS files
○ scripts/shared.js => shared code that runs on both pages
○ scripts /main.js => code that runs on index.html
○ scripts /view.js => code that runs on view.html
● One JS Library file
○ libs/jscolor.js => 'jscolor' library for color picker
Please follow the order of tasks provided as it will make developing and completing this topic easier.
Provided Local Storage Code
Here, we have provided some code to ensure that the data in the TaskList class instance task is persistent in the browser.
1. We have defined some constant variables for you, which are the two KEYS that you will use for storing data in local storage. These are called
TASK_INDEX_KEY and LIST_DATA_KEY.
2. We have defined a global TaskList instance variable to use throughout the app, tasks.
3. The function checkIfDataExistsLocalStorage is responsible for checking to see if data exists in local storage at the defined key.
The way this works is to first retrieve the data at the key TASK_DATA_KEY and then check to see if it:
。 exists
。 contains null
。 contains undefined
。 contains a blank string
4. If it exists and doesn't contain any of the above, then the function will return true. Otherwise, it will return false.
5. The function updateLocalStorage has one parameter, data.
This function is responsible for storing the provided data in local storage at the key TASK_DATA_KEY.
This is done by stringifying the data, then storing it in local storage using the key TASK_DATA_KEY.
We will call this function whenever we make any changes to the data, and will use it to update the 'backup' in local storage.
6. The function getDataLocalStorage is responsible for retrieving data from local storage at the key TASK_DATA_KEY.
This is done by retrieving the data using the key TASK_DATA_KEY, parsing it back into an object, then returning it.
7. Global code that runs when the page loads.
When the page loads,we check if data exists at the key TASK_DATA_KEY in local storage.
If data exists, we retrieve it using getDataLocalStorage and restore the data into the global TaskList instance variable tasks.
If it doesn't exist, then we add a single task with the ID "Task1", and update local storage with the TaskList.
Working on shared.js
Task 1 involves shared.js
Task 1: Writing Classes
In this task, we will be writing the classes which form the backbone of the app, and be responsible for storing all data about each task and list of tasks.
All code in this task should be written in shared.js.
1. Write code necessary to implement the two classes shown in the class diagram.
Here's some additional information regarding the methods of the TaskList class. 。 get count() accessor
This accessor should return the length of the _tasks array (i.e the number of tasks currently in the list)
。 addTask method
This method has two parameters, id and pin.
It should create a new instance of Task using the id and the pin
provided, and add it to the _tasks array.
。 getTask method
This method has one parameter, index.
It should return the Task at index in the _tasks array.
。 removeTask method
This method has one parameter, id.
It should remove the Task with the id from the _tasks array using splice.
。 Refer to the reading material on 7.1 Local Storage (last two pages) for the fromData method.
。 The constructor for the Task class takes two parameters, id and pin.
。 The constructor for the TaskList class has no parameters.
Working on main.js and index.html
Tasks 2 - 4 involve main.js and index.html
Task 2: Display All Tasks
Here, we will write some code to display all the tasks graphically on the HTML page.
All code in this task should be written in main.js.
1. Take a look in the main.js file. Lines 14 to 48 contain some JS code for a single task. For this step, you need to use this code to generate a task for each task in the global TaskList instance variable, tasks.
2. Write the function displayTasks.
Note: We have already filled this function with code to generate 1 generic task which is displayed on the page. You will need to use this code to generate
multiple tasks based on the data.
This function should take one parameter, data.
This function is responsible for generating the HTML code required for all the
tasks. The HTML generated should be displayed in the div with ID taskDisplay.
You should also ensure that the task is displayed under relevant heading according to their status (to do, in progress or completed).
Also, each task’s locked status must be shown as well using the material icon lock with open_Lock for an open task and closed_Lock for a closed task.
Note: The task display should also have the option to delete the task by
clicking on the rubbish bin icon. It will be implemented as a separate function.
You should begin by creating an output variable, and looping over all the tasks in the TaskList instance (which is passed to this function as a
parameter), and generate the appropriate HTML with the values from each task, then display it on the page using the innerHTML property of the
taskDisplay div.
Hint: We recommend you use the concatenation operator += to append to the output variable and only set the innerHTML property once outside the loop.
3. Next, write some code that will run on page load
You should call the function displayTasks on page load, and pass it the global tasks as a parameter.
Task 3: Add a New Task
Here you will write the function to add a new task to the TaskList instance.
All code in this task should be written in main.js.
1. Write a function addNewTask
This function is responsible for the interaction with the user to create a new task using the addTask method in the TaskList class.
Task 4: View Task Information
Here you will write the function that is responsible for storing some necessary information, then redirecting the user to the view page.
All code in this task should be written in main.js
1. Write the function view
This function has one parameter, index where index is the index of the task.
This function stores the tasks index into localStorage and redirects the user to view.html to view the task.
Note: You can redirect a user by using window.location like this:
window.location = "nextpage.html";
Working on view.html and view.js
Tasks 5 - 7 involve view.html and view.js
Task 5: Displaying Task Information
The view page is used to display information and actions for a specific task. To that end, we previously stored the index of the selected task in local storage using the key TASK_INDEX_KEY.
Take a look at view.html. This file has some placeholders for HTML code.
1. You will need to write some HTML code in view.html using MDL [link]for the following:
○ "Content" that contains
■ H4 header with text "Description"
■ textarea with id "taskDescription" to hold the description text of the task
○ "Task Settings area" that contains
■ H4 header with text "Settings"
■ MDL input field with id "taskLabel" to hold the label text of the task
■ MDL input field with id "taskColor" to hold the color of the task
○ “Task lock Setting” area with radio buttons defined as
■ H4 header with text “Task Status”
■ MDL radio button with text "To Do" and default checked
■ MDL radio button with text "In progress"
■ MDL radio button with text "Completed"
○ Buttons defined as
■ MDL button (blue, ripple) with text "Update Task” and onclick "upDatetaskDetails()"
■ MDL button (pink, ripple) with text "Lock Task" and onclick "lockTask()"
2. Note that we will be using the jscolor library, which adds a color picker to an input field.
To activate this element, you need to add the jscolor class to the input
element for taskColor.
Note: Refer to this link for more detail https://jscolor.com/
3. Next, in view.js, write the function displayTaskInfo.
This function takes one parameter, task.
It is responsible for displaying / populating the task information into the HTML page (using DOM Manipulation).
You should ensure that you display the information of the task provided in the parameter, including its id, label, color, description and status.
4. In view.js, write a function updateTaskDetails().
It is responsible for changing the task details such as description, new label and status,
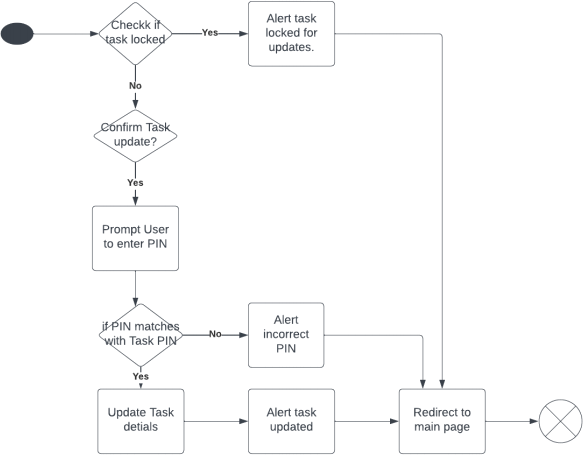
5. If the task is locked then the task is locked for updates and an appropriate alert message is displayed to inform the user that the task is locked and no updates can be performed.
6. Finally, in view.js, write some code that executes when the page loads.
The code should retrieve the stored index of the selected task from local
storage at key TASK_INDEX_KEY, and then retrieve the Task instance using the method getTask. And finally call displayTaskInfo function to display the
information about the selected Task instance stored in the variable task.
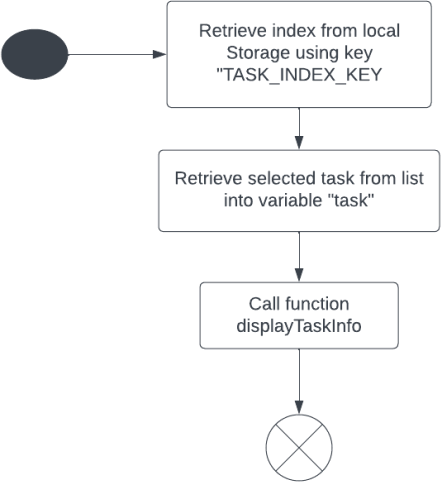
Task 6: Delete Task
On the view page, we can delete the current task (if you want to do so).
All code for this task should be written in view.js.
1. Write the function deleteThisTask.
The function should delete the task at the relevant index and then update local storage by calling updateLocalStorage.
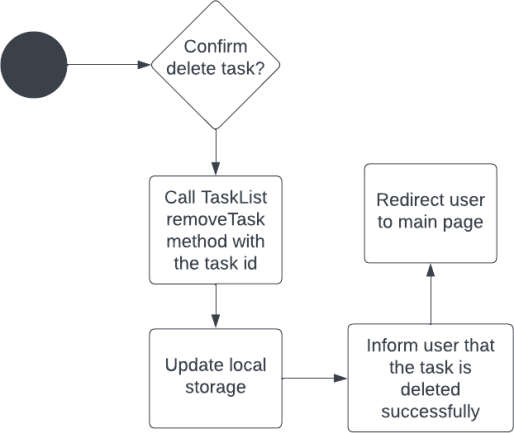
Hint: You can ask the user to confirm something by using the confirm() function (in- built JS).
You can inform the user of something by using the alert() function (in-built JS).
// Confirm with the user
if (confirm("Are you very sure?"))
{
// runs if user clicks 'OK'
}
else
{
// runs if user clicks 'Cancel'
}
2. Modify the displayTaskInfo function you wrote earlier, and add an event
listener for the click event to the delete button on the view page (Line 26). The delete button has been defined for you with the ID deleteTask
The event listener should execute the function deleteThisTask.
Task 7: Lock the Task
On the view page, we can lock the task when we are done.
All code for this task should be written in view.js.
1. Write the function lockTask.
Finally, clean up your code and make sure you meet the requirements for:
● Having appropriate code Indentation and Code Brackets (per the style guide)
● Having appropriate variable and function naming (per the style guide)
● Having appropriate variable and function scoping (smallest possible)
● Having appropriate function header documentation (name, purpose of function, parameter and return value description)
● No use of magic numbers
2023-08-19