Final Project: Disaster Relief Robots
Hello, dear friend, you can consult us at any time if you have any questions, add WeChat: daixieit
Final Project: Disaster Relief Robots
Introduction
You are a software developer at RescueBots Inc., a robotics company that develops technology to aid in life-threatening situations. The company has recently built a leet of autonomous robots that can assist in rescue operations during natural disasters, such as bushires and loods. RescueBots' robots can navigate unknown terrain and successfully locate and rescue people in need of help. For these robots to be truly autonomous and efective, however, they must be capable of making diicult decisions. The RescueBots robots must be programmed to prioritize and determine the order of rescue operations, a feat with ethical and moral implications.
In this project, you will create the decision engine for RescueBots, i.e., a program designed to explore diferent scenarios and make critical decisions about whom to save. You will inally audit your decision-making algorithm through simulations, and allow users of your program to judge the outcomes themselves.
Academic Honesty
All assessment items (assignments, test and inal project) must be your own, individual, original work.
Any code that is submitted for assessment will be automatically compared against other students' code and other code sources using sophisticated similarity checking software.
Cases of potential copying or submitting code that is not your own may lead to a formal academic misconduct hearing and prosecution.
Potential penalties can include getting zero for the project, failing the subject, and even expulsion from the university in extreme cases.
For further information, please see the university's Academic Integrity website or ask your teaching team.
Pre-amble: Object Oriented Design
In this inal project, you will need to follow best practices of Object-Oriented Programming as taught and discussed throughout the semester.
When creating packages and classes, carefully consider which members (classes, instance variables or methods) should go where, and ensure you are properly using polymorphism, inheritance, and encapsulation in your code.
One class has been provided to you in the starter code (RescueBot.java), which must be used as your program's entry point. You will need to add additional classes and possibly a package so as to make your code more modular.
This project requires a signiicant amount of time so make sure you plan accordingly! Time management is of the essence when developing software. Start early, don't leave it to the last week to ire up your IDE!
Ready. Steady. All the best!
Project Setup
Program Launch with Flags
We will use command-line options or so-called ags to initialize the execution of RescueBot. Therefore, you need to add a few more options as possible command-line arguments.
Hence, your program is run as follows:
? t6v6 H92ou9:o才 ]6含3um9n才2[
Print Help
Make sure your program provides a help documentation to tell users how to correctly call and execute your program. The help is a printout on the console telling users about each option that your program supports.
The following program calls should invoke the help:
? t6v6
o含
? t6v6
H92ou9:o才 --n9Jq
H92ou9:o才 -n
The command-line output following the invocation of the help should look like this:
H92ou9:o才 - )0Mqe00卜上 - 刁rn6J q含ot9o才
U2639: t6v6 H92ou9:o才 ]6含3um9n才2[
A含3um9n才2:
-2 o含 --2o9n6含ro2
-n o含 --n9Jq
-J o含 --Jo3
The help should be displayed when the --help or -h lag is set or if the --scenarios or --log lag is set without an argument (i.e., no path is provided). The lag --scenario or -s tells your program about the path and ilename of the ile that contains predeined scenarios. The lag --log or -l indicates the path and ilename where scenarios and user judgements should be saved to. More about that later.
Multiple lags can be used and their order is interchangeable, for example:
? t6v6 H92ou9:o才 -2 2o9n6含ro2 .o2v -J Jo3个rJ9 .Jo3
$ java RescueBot -l logfile .log -s scenarios .csv
The help should always be displayed when your program is invoked with an invalid set of arguments.
The Class RescueBot.java
This class provided in the code skeleton holds the main method and manages your program execution. It needs to take care of the program parameters as described above.
This class also houses the decide method, which implements the decision-making algorithm.
Decision Algorithm
Your task is to implement the public static method decide(Scenario scenario), which returns an object of the type Location, which will be speciied later. Your code must choose a location that contains a group of characters that will be saved for the given scenario.
To make the decision, your algorithm needs to consider the attributes of the characters involved as well as the situation. You can take any of the characters' characteristics (age, body type, profession, etc.) into account when making your decision, but you must base your decision on at least 5 characteristics–from the scenario itself (e.g., whether the characters have illegally trespassed) or from the characters' attributes. Note that there is no right or wrong in how you design your algorithm. Execution is what matters here so make sure your code meets the technical speciications. But you may want to think about the consequences of your algorithmic design choices.
Main Menu
Once the program is launched, the main menu is shown with a welcome screen: your program should read in and display the contents of welcome.ascii to the user without modifying it. The message provides background information about RescueBot and walks the user through the program low.
The main menu will form the core of your system and will control the overall low of your program. When your program is run, it should show the following output:
$ javac * .java
$ java RescueBot -s scenarios .csv
__
_ (\ |@@|
(__/\__ \--/ __
\___ |---- | | __
\ }{ /\ )_ / _\
/\__/\ \__O (_COMP90041
(--/\--) \__/
_)( )(_
`--- ''--- `
$$$$$$$\ $$$$$$$\ $$\
$$ __$$\ $$ __$$\ $$ |
$$ | $$ | $$$$$$\ $$$$$$$\ $$$$$$$\ $$\ $$\ $$$$$$\ $$ | $$ | $$$$$$\ $$$$$$\ $$$$$$$ |$$ __$$\ $$ _____ |$$ _____ |$$ | $$ |$$ __$$\ $$$$$$$\ |$$ __$$\\_$$ _ |
$$ __$$< $$$$$$$$ |\$$$$$$\ $$ / $$ | $$ |$$$$$$$$ |$$ __$$\ $$ / $$ | $$ |
$$ | $$ |$$ ____ | \____$$\ $$ | $$ | $$ |$$ ____ |$$ | $$ |$$ | $$ | $$ |$$\
$$ | $$ |\$$$$$$$\ $$$$$$$ |\$$$$$$$\ \$$$$$$ |\$$$$$$$\ $$$$$$$ |\$$$$$$ | \$$$$ | \__ | \__ | \_______ |\_______/ \_______ | \______/ \_______ |\_______/ \______/ \____/
Welcome to RescueBot!
The idea of RescueBot is based on the Trolley Dilemma, a fictional scenario presenting a decision m
The answers are not straightforward . There are a number of variables at play, which influence how p
{N} scenarios imported .
Please enter one of the following commands to continue:
- judge scenarios: [judge] or [j]
- run simulations with the in-built decision algorithm: [run] or [r]
- show audit from history: [audit] or [a]
- quit the program: [quit] or [q] >
This initial output is made of two parts:
1. The welcome text, which you need from the ile provided to you in the starter code
2. The number of scenarios that were imported from the scenarios ile. {N} depicts the number. If no scenarios ile is provided at program launch, this line should be removed.
3. An initial message explaining how the user should use the terminal, followed by a command prompt "> ", which waits for the user to enter a command and hit return to continue.
Here is where the user selects an option for the program execution. If the user enters a command that is not listed in the menu, the following message should be output, followed by the menu prompt:
Invalid command! Please enter one of the following commands to continue:
- judge scenarios: [judge] or [j]
- run simulations with the in-built decision algorithm: [run] or [r]
- show audit from history: [audit] or [a]
- quit the program: [quit] or [q] >
Reading in Scenarios
Your program needs to support the import of scenarios from a scenarios ile. The path to the scenarios ile is optionally provided as a command-line argument when running your program:
$ java RescueBot --scenarios path/to/scenarios .csv
or
$ java RescueBot -s path/to/scenarios .csv
These program calls above are equivalent and should both be supported by your program.
The command-line argument following the lag --scenarios or -s respectively speciies the ile path where the scenarios ile (scenarios.csv in this case) is located. Your program should check whether the ile is located at the speciied location and handle a FileNotFoundException in case the ile does not exist. In this case, your program should terminate with the following error message:
java.io.FileNotFoundException: could not find scenarios file .
If no scenarios argument is provided, your program needs to create its own scenarios randomly.
Parsing the Scenarios File
Next, your program needs to read in the scenarios ile. The Table below lists the contents of the provided scenarios.csv, a so-called comma-separated values (CSV) ile. The ile contains a list of values, each separated by a comma.
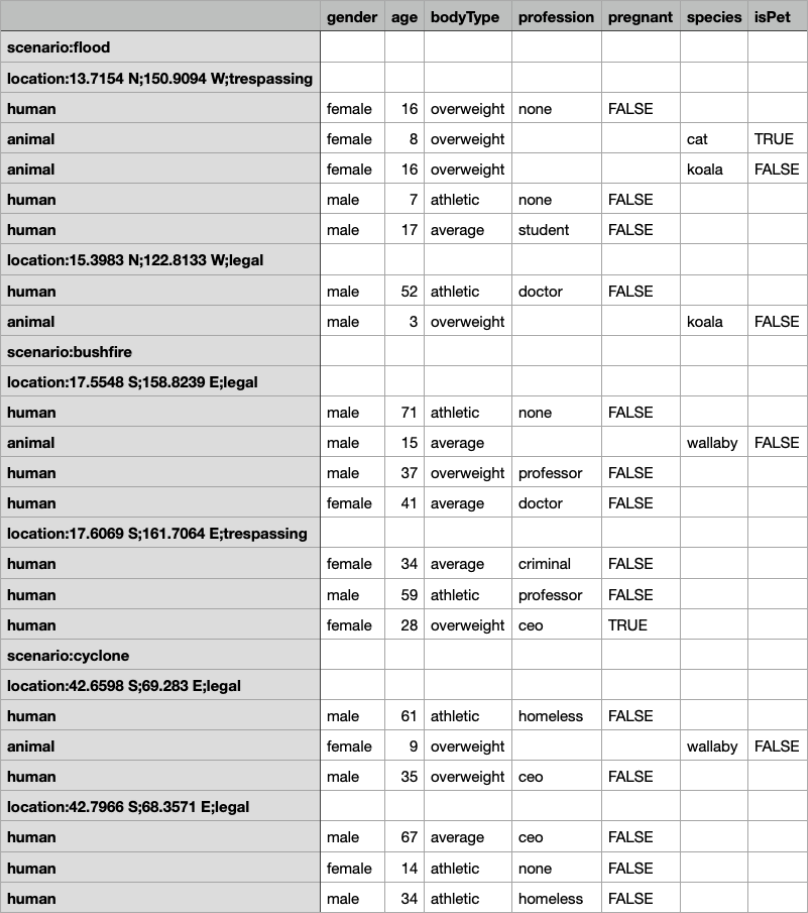
As seen in the Table, the irst line contains the headers, i.e., the names of each data ield and can therefore be ignored by your program. Each subsequent row presents an instance of a scenario, location, or character. Scenarios are preceded by a single line that starts with scenario: followed by the descriptor (e.g., lood, bushire, etc.). For each scenario, there are multiple locations, in which a group of characters are awaiting rescue. The table lists each location as location: followed by the location's latitude, longitude, and whether the characters are trespassing or have legally entered the location.
In the provided scenarios.csv, the irst scenario describes a lood with characters trapped in 2 locations. The irst group is trespassing and is made up of 3 humans and 2 animals. The second group has legally entered a location nearby and is made up of one human doctor and one koala.
There are two more scenarios depicted in this ile, a bushire and a cyclone event.
Your RescueBot class needs to be able to parse the scenarios ile and create scenarios for the user judgement or simulation. Note that a scenarios ile can contain any number of scenarios with any number of locations and characters. You can assume that all scenarios iles follow the same column order as shown in the Table.
You will be provided with several scenarios iles for testing purposes.
Handling Invalid Data Rows
While reading in the scenarios ile line by line your program may encounter three types of exceptions, which your program should be able to handle:
1. Invalid Data Format
You need to handle exceptional cases where there is an invalid number of data ields per row: in case the number of values in one row is less than or exceeds 8 values an InvalidDataFormatException should be thrown. Your program should handle such exceptions by issuing the following warning statement to the command line, skip the respective row, and continue reading in the next line.
WARNING: invalid data format in scenarios file in line {X}
2. Invalid Number Format
This refers to an invalid data type in a cell: in case the value can not be cast into an existing data type (e.g., a character where an int should be for age) a NumberFormatException should be thrown. More about valid characteristics later.
Your program should handle such exceptions by issuing the following warning statement to the command line, assign a default value instead, and continue with the next value in that line.
WARNING: invalid number format in scenarios file in line {X}
3. Invalid Field Values
In case your program does not accommodate a speciic value (e.g., skinny as a bodyType) an InvalidCharacteristicException should be thrown. Your program should handle such exceptions by issuing the following warning statement to the command line, assign a default value instead, and continue with the next value in that line.
WARNING: invalid characteristic in scenarios file in line {X}
Note that {X} depicts the line number in the scenarios ile where the error was found.
The warnings above should be printed to the console in the order they are encountered after the welcome message as shown below:
__
_ (\ |@@|
(__/\__ \--/ __
\___ |---- | | __
\ }{ /\ )_ / _\
/\__/\ \__O (_COMP90041
(--/\--) \__/
_)( )(_
`--- ''--- `
$$$$$$$\ $$$$$$$\ $$\
$$ __$$\ $$ __$$\ $$
$$ | $$ | $$$$$$\ $$$$$$$\ $$$$$$$\ $$\ $$\ $$$$$$\ $$ | $$ | $$$$$$\ $$$$$$\ $$$$$$$ |$$ __$$\ $$ _____ |$$ _____ |$$ | $$ |$$ __$$\ $$$$$$$\ |$$ __$$\\_$$ _ |
$$ __$$< $$$$$$$$ |\$$$$$$\ $$ / $$ | $$ |$$$$$$$$ |$$ __$$\ $$ / $$ | $$
$$ | $$ |$$ ____ | \____$$\ $$ | $$ | $$ |$$ ____ |$$ | $$ |$$ | $$ | $$ |$$\
$$ | $$ |\$$$$$$$\ $$$$$$$ |\$$$$$$$\ \$$$$$$ |\$$$$$$$\ $$$$$$$ |\$$$$$$ | \$$$$ | \__ | \__ | \_______ |\_______/ \_______ | \______/ \_______ |\_______/ \______/ \____/
Welcome to RescueBot!
The idea of RescueBot is based on the Trolley Dilemma, a fictional scenario presenting a decision m The answers are not straightforward . There are a number of variables at play, which influence how p
WARNING: invalid characteristic in scenarios file in line 7
3 scenarios imported .
Please enter one of the following commands to continue:
- judge scenarios: [judge] or [j]
- run simulations with the in-built decision algorithm: [run] or [r]
- show audit from history: [audit] or [a]
- quit the program: [quit] or [q]
>
Judging Scenarios
When a user selects [judge] or [j] from the main menu, the following program sequence should execute:
1. Collect user consent for saving data
2. Present scenarios from scenarios ile or randomly generated
3. Show statistic
4. Save judged scenarios and decisions (if permissible)
5. Repeat or return: show more scenarios or return to the main menu
1. Collect User Consent
First, your program should collect the user's consent before saving any results. Explicit consent is crucial to make sure users are aware of any type of data collection. Your program should, therefore, ask for explicit user consent before logging any user responses to a ile. Before showing the irst scenario, your program should, therefore, prompt the user with the following question on the command line:
Do you consent to have your decisions saved to a file? (yes/no)
>
Only if the user conirms (yes), your program should save both the scenario and the user's judgement to the log ile (if the ilename is not explicitly set by the command-line lag (--log) save the results to the ile rescuebot.log in the default folder. If the user selects no, your program should function normally but not write any of the users' decisions to the ile (it should still display the statistic on the command line though). If the user types in anything other than yes or no, an InvalidInputException should be thrown and the user should be prompted again by displaying:
Invalid response! Do you consent to have your decisions saved to a file? (yes/no) >
2. Present Scenarios
Once the user consented (or not), the scenario judging begins. Therefore, scenarios are either imported from the scenarios ile or (if the scenarios ile is not speciied) randomly generated.
A scenario contains all relevant information about the type of scenario, the locations, and the characters therein. Here is an example:
======================================
# Scenario: flood
======================================
[1] Location: 13.7154 N, 150.9094 W
Trespassing: yes
5 Characters:
- overweight child female
- cat is pet
- koala
- athletic child male
- average adult student male
[2] Location: 15.3983 N, 122.8133 W
Trespassing: no
2 Characters:
- athletic adult doctor male
- koala
To which location should RescueBot be deployed?
>
Each location is numbered in ascending order. The user picks the location where RescueBot should be deployed by entering the corresponding number, which results in the saving of the characters in that location.
Characters' attributes are written in lowercase and separated by single space. Your output must match the output speciications as described below:
Character Attributes
Characters in a scenario are either humans or animals. They share some traits and difer in others. The following are some shared attributes you need to take into account:
each character has an age, which should be treated as a class invariant for which the following statement always yields true: age >= 0.
gender: must at least include the values FEMALE and MALE as well as a default option UNKNOWN, but can also cover more diverse options if you so choose.
bodyType: must be made up of the values AVERAGE, ATHLETIC, and OVERWEIGHT as well as a default option UNSPECIFIED.
Humans
More speciically, scenarios are inhabited by humans who exhibit the following characteristics:
ageCategory: depending on the age, each human should be categorized into one of the following:
BABY: a human with an age between 0 and 4.
CHILD: a human with an age between 5 and 16.
ADULT: a human with an age between 17 and 68.
SENIOR: a human with an age above 68.
humans tend to have a profession. In these scenarios, you should include the following values:
DOCTOR
CEO
CRIMINAL
HOMELESS
UNEMPLOYED
NONE as default
You can add your own professions as well.
Note that only ADULTs have professions, other age categories should be classiied as NONE. Additionally, you are tasked with coming up with at least two more profession categories you deem feasible.
Furthermore, female humans can be pregnant. Think of it as another class invariant where males cannot be pregnant and only ADULTfemales should be able to.
Humans have a speciic output format when printed to the command line. Regardless of whether you add any custom characteristics or not, in a given scenario, humans must be described as follows:
Note that attributes in brackets [] should only be shown if they apply, \textit{e.g.}, a baby does not have a profession so, therefore, the profession is not displayed.
Here is an example:
athletic adult doctor female
or
average adult doctor female pregnant
Note that words are in lowercase and separated by single spaces. Age, other than age category, is ignored in the output.
Animals
At last, animals are part of the environment we live in. People walk their pets so make sure your program accounts for these. Animals share some characteristics with humans, but also have the following speciic attributes:
species: this indicates what type of species the animal represents, for example, a koala or dog. Make sure your program accounts for arbitrary animal species. You can also invent your own. isPet: animals can be pets. If so, it needs to be mentioned in the scenario.
Animals also have a speciic output format when printed to the command line, which should follow the following speciication:
Note that only dogs, cats, and ferrets can be pets but don't need to be.
Here is a concrete example:
cat is pet
Here is another example where the animal is not a pet:
platypus
Note that words are in lowercase, separated by single spaces, and that age, gender, and bodyType are ignored in the animal's output.
Scenario Attributes
Scenarios list the diferent locations, in which groups of humans and animals gather. Each location has a latitude, longitude, and the information whether the characters have trespassed or legally entered the area. When printed to the command line a scenario should follow this format:
======================================
# Scenario:
======================================
[N] Location:
Trespassing: {yes, no}
{# of characters} Characters:
-
.
.
.
[N+1] Location:
Trespassing: {yes, no}
{# of characters} Characters:
-
.
.
.
Your output must match these output speciications.
For user judgement, scenarios are presented on the command line one by one as described. Each scenario should be followed by the following prompt:
To which location should RescueBot be deployed?
>
If the user enters anything but a valid location number, the following message should be displayed before awaiting a new user response:
Invalid response! To which location should RescueBot be deployed?
>
After the user made a decision, the next scenario is shown, followed by yet another prompt to judge the scenario. This procedure should repeat until 3 scenarios have been shown and judged. After the third scenario decision, the result statistic is presented.
Random Scenario Generation
If no scenario ile is provided when your program is executed, you need to generate them randomly. To guarantee a balanced set of scenarios, it is crucial to randomize as many elements as possible, including the number and characteristics of humans and animals involved in each scenario as well as the locations (i.e., valid latitudes, longitudes, and whether groups have been trespassing).
The minimum number of locations for each scenario should be 2. No maximum needed. At least one human or animal should be present in each location.
2023-06-09